The Future is
Remocal*
mirrord solves the problems every modern cloud developer faces during microservice development by making remote services feel local.
*What’s Remocal?
*What’s Remocal?
Remote + Local = Remocal.
mirrord makes your local development experience feel 100% remote by making the running process think it's running in Staging. That means when you run your code with mirrord, it's the same as running it in Staging, without having to move your code around, 'git push' to test, or do anything more than work directly from your CLI or IDE as you always have.


Modern Cloud Developers Deserve Modern Tools
mirrord for Teams helps developers stay local, ship code confidently, and makes precious staging envs a thing of the past

Stay Local
The mirrord CLI, VSCode and IntelliJ Plugins mean that they can embrace the Remocal Future and experience modern development with mirrord for Teams from the comfort of their current setup.

Staging for everyone
mirrord for Teams lets developers concurrently access staging resources throughout the entire development process, not just when the code is “ready for Staging.”
Put an end to `git push` testing
When a typical cloud developer wants to see how their work will perform in staging and production, they `git push`, trigger CI/CD, and wait. And wait – and this kills progress and innovation.

From cloning a microservice repo to developing against a fully functional staging environment in minutes
Better access to real environments means that mirrord for Teams helps you help your developers ship more code easily and securely.
`brew install` and that’s it.
Fire up your service from your laptop, and it will act 100% like it is running in k8s in staging – mirrord makes getting started contributing to a complex services-based Kubernetes architecture as easy as cloning a repo and installing a CLI tool (or IDE extension!).
Nothing extra to manage.
mirrord works on the principle of “setup once, and love forever.” Once a service is running with mirrord, everyone who can clone the repo will automatically enjoy the benefits of using the service Remocally.
Access to remote *everything*
mirrord is easy to explain but hard to implement – and it works like magic. Cloud developers can fire up the CLI tool and develop against full staging traffic, disks, queues, DNS, and more – as if they had the full Staging cluster running on their local machine.
From solo developers to large Enterprises, mirrord is helping usher in the modern developer experience
Private, secure, and designed to keep your data where it belongs – mirrord for Teams is built for companies of any size.
EXPERTS

Built by experts
mirrord is built by a team of k8s experts who have made a tool beloved in the Open Source community, and now they’re bringing their expertise to the Enterprise.
SIMPLE

Built for simplicity
mirrord for Teams lets your team concurrently work on the same pods, use RBAC to keep access tightly controlled, and more – without a bunch of extra stuff to manage.
ENTERPRISES

Built for Enterprises
mirrord for Teams fits in larger, security sensitive Enterprises. Rest assured knowing that nothing leaves your environment, nothing ever hits our servers, and your data is yours alone.
We’re holding a mirror up to our awesome community
Check out what folks have to say about using mirrord for Remocal development
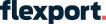
“[mirrord] has significantly improved our development experience, making the team happier and more efficient.”
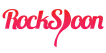
“We are using mirrord... to debug issues and test local changes, in our shared staging environment, without impacting other developers. ”
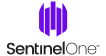
“Instead of asking every developer to create and fill a local environment, we have a single large remote one that is used by everyone.”
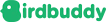
“[With mirrord] the service is running locally (fast iterations) but still receives all the cluster access including databases, credentials, configuration.”
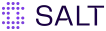
“With the use of mirrord and its extensions, we were able to [debug a complete workflow], significantly improving the development feedback cycle.”

Learn More About Remocal Development
The funny-sounding buzzword that is changing how developers work – forever.
*What’s Remocal?
Remote + Local = Remocal.
mirrord makes your local development experience feel 100% remote by making the running process think it's running in Staging. That means when you run your code with mirrord, it's the same as running it in Staging, without having to move your code around, 'git push' to test, or do anything more than work directly from your CLI or IDE as you always have.
